Go for Gold: A Classic Warm-Up Exercise
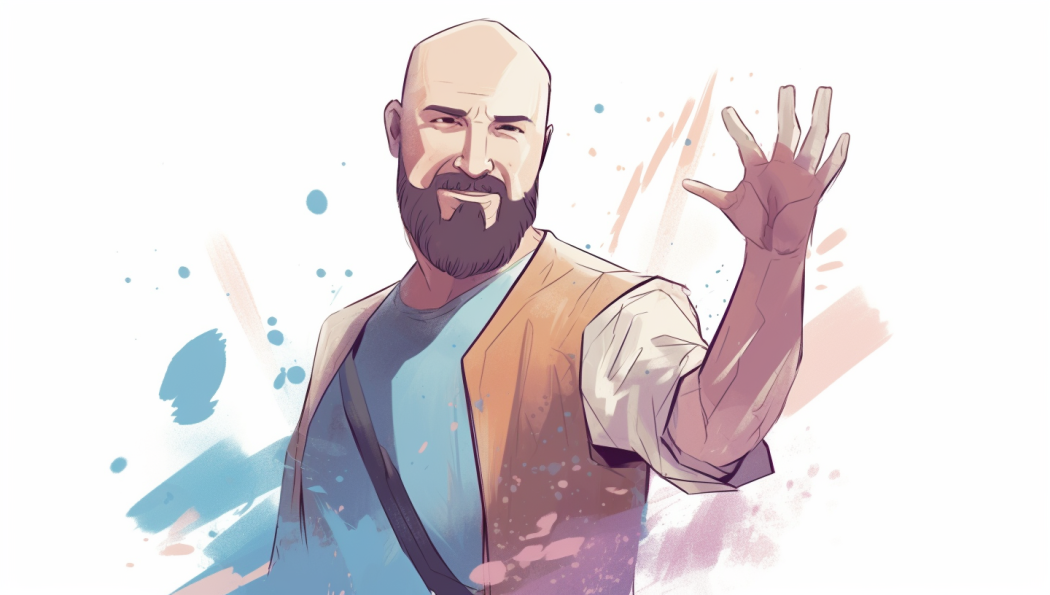
Prerequisites
If you haven't installed Go yet, don't worry. Here's a helpful guide to get Go up and running on your system: https://go.dev/doc/install
Development Environment
For people who write code, choosing a tool to write in is super important. There are lots of free tools, like Visual Studio Code, NeoVim, and NeoVim that are really good. Right now, I like using NeoVim the most and want to make it just right for me.
I'm making a simple guide to set up NeoVim, Emacs, and Visual Studio Code. If you can’t wait, check out NvChad, SpaceMacs and DoomEmacs. They do a lot of the setup work for you! (You’ll still need to do a bit more setup if you're using them for Go programming.)
But if you're brand new to coding and have never used those other console-based editors, it’s best to stick with Visual Studio Code. This way, you don’t have to learn a new way to edit text at the same time as learning a new programming language.
Check out this guide.
Creating a Project
Remote repo
Now that we've covered Go installation and configured our development environment, let's move on to the next step.
Let's create a remote repository on GitHub, they provide a comprehensive step-by-step guide to help you get started.
Initializing the Project
To keep your projects organized, consider creating a dedicated directory.
- On Mac or Linux, you can use
~/Projects
. - On Windows, use
C:\Users\YourUsername\Projects
.
$ cd ~/Projects
$ md plykan
$ go mod init github.com/solutioncrafting/plykan
Henceforth, we'll assume our projects are located at ~/Projects
for simplicity.
Push the changes
$ git init
$ git add .
$ git commit -m "Initial commit"
$ git remote add origin git@github.com:solutioncrafting/plykan.git
Note: In the examples provided, make sure to replace the URLs with your own repository URLs.
We are ready to start coding.
Routing 101: Linking URLs with Handlers
Key Concepts:
- URL: The designated pathway that leads to a specific action in your app.
- Handler: Consider it your go-to worker. For now, it manages tasks, but over time, its role will shift towards coordinating.
- Router: The vital link between a URL and its Handler.
- Server: The gateway that presents the router’s associations to the broader world.
On a more abstract level:
- Request: In the realm of web apps, think of this as a plea to a server, seeking information or action.
- Response: The server’s retort, either offering the data you asked for or confirming that the task was done.
In Go, both "Request" and "Response" are not just abstract ideas; they have tangible representations as a struct and an interface, respectively. We'll delve into this deeper later on.
Go's standard library equips you with all the tools needed to craft a web application without leaning on external dependencies.
This includes servers and routers, and many of their functions come via specific interface implementations defined within the library.
Although the Go standard library is robust and comprehensive, it's worth mentioning that alternative implementations are also widely used. These libraries are favored for their ability to reduce the boilerplate required for feature implementation and enhance performance.
We'll be harnessing a mix. We will rely on well-known libraries and toolkits if they favor the efficient development of our goals. However, when it's valuable to understand the foundational basics, we'll touch upon vanilla stdlib implementations. In such instances, a link to a side article will be made available.
For this project, the election falls on the Gorilla web toolkit. Its creators describe it as a valuable toolkit for the Go programming language, offering convenient packages designed for crafting HTTP-based applications.
package main
import (
"fmt" // 1
"net/http"
"github.com/gorilla/mux" // 2
)
func main() {
r := mux.NewRouter() // 3
r.HandleFunc("/hello", handler) // 4
http.Handle("/", r) // 5
fmt.Println("Starting server...") // 6
err := http.ListenAndServe(":8080", nil) // 7
if err != nil { // 7
fmt.Printf("Server error: %s\n", err) // 8
}
}
func handler(w http.ResponseWriter, r *http.Request) {
_, err := fmt.Fprintf(w, "Hello, World!") // 9
if err != nil {
return // 10
}
}
https://github.com/solutioncrafting/hello/blob/main/main.go
Hello, World!
Let's develop our first web app
Code Glimpse
- We import standard library packages like
fmt
andnet/http
for formatting and to leverage HTTP server implementations. - We also bring in the
gorilla/mux
package for its advanced router, which helps associate different handler functions with different routes. - In the
main
function, we create an instance of a mux router. This is the reason for importing thegorilla/mux
package. - Using
HandleFunc
, we associate the/hello
route with thehandler
function, setting it up to handle requests to this specific route. - http.Handle("/", r) allows our router to manage all incoming HTTP requests to the server.
- Before the server starts, we print "Starting server..." to the console as a friendly heads-up that it’s about to get going.
- We initialize the server to listen on port 8080 with http.ListenAndServe(":8080", nil), standing by for incoming connections.
- If there’s an error during server initialization, it's promptly printed to the console to keep us informed.
- We define a handler function to respond to any requests made to the "/hello" route, writing "Hello, World!" to the HTTP
ResponseWriter
. - When writing the response, if any error occurs, it's gracefully handled without causing a disruption.
I recommend checking out the GitHub repository for this and future code. There, In the repo, all comments are directly linked to the code they explain, making it clearer than it would be here in the post. I avoid adding those comments here to keep the post clean and clear.
Running the app
$ go run main.go
Open your browser and go to http://localhost:8080/hello
You should see something like this

As we wrap up this initial step of creating a simple web app, I know seasoned Go developers might find this a bit basic or slow-paced. Bear with me, this is just the foundation, and things will soon get more exciting and we’ll pick up the pace, taking a more direct approach.
Stick around and follow this series. To get the newest posts straight to your inbox, consider subscribing to our newsletter.
Up Next: Routing
In our next post, we will cover the topic of Routing in depth. We'll detail the process of creating RESTful routes and discuss the further development of our application based on the Kanban board system. Be sure to check it out for a comprehensive overview of these updates.
See you!
References
Previous article: 1 - Exploring Real-World Go Application Development